高分求c#方法
用c#写一个函数,要求:1、传入参数开始日期,结束日期,每天开始营业时间,结束营业时间,整数N
2、随机产生N个时间点,并返回
3、产生的时间点必须是在营业时间内的,举例:
10月1日 - 10月3日, 9:00 - 22:00,
调用函数的时间是10月1日 12:00分,那么随机产生的时间点范围肯定在
10月1日 12:00 - 22:00
10月2日 09:00 - 22:00
10月2日 09:00 - 22:00
3个区间以内
4、返回的时间点按从小到大排序返回
--------------------编程问答-------------------- 如何处理datetime的问题,可以msdn http://msdn.microsoft.com/en-us/library/system.datetime.aspx --------------------编程问答--------------------
using System;
namespace WindowsApplication2
{
public class DateTimeHelper
{
/**/
/// <summary>
/// 获取随机时间
/// <remarks>
/// 由于Random 以当前系统时间做为种值,所以当快速运行多次该方法所得到的结果可能相同,
/// 这时,您应该在外部初始化 Random 实例并调用 GetRandomTime(DateTime time1, DateTime time2, Random random)
/// </remarks>
/// </summary>
/// <param name="time1"></param>
/// <param name="time2"></param>
/// <returns></returns>
public static DateTime GetRandomTime(DateTime time1, DateTime time2)
{
Random random = new Random();
return GetRandomTime(time1, time2, random);
}
/**/
/// <summary>
/// 获取随机时间
/// </summary>
/// <param name="time1"></param>
/// <param name="time2"></param>
/// <param name="random"></param>
/// <returns></returns>
public static DateTime GetRandomTime(DateTime time1, DateTime time2, Random random)
{
DateTime minTime = new DateTime();
DateTime maxTime = new DateTime();
System.TimeSpan ts = new System.TimeSpan(time1.Ticks - time2.Ticks);
// 获取两个时间相隔的秒数
double dTotalSecontds = ts.TotalSeconds;
int iTotalSecontds = 0;
if (dTotalSecontds > System.Int32.MaxValue)
{
iTotalSecontds = System.Int32.MaxValue;
}
else if (dTotalSecontds < System.Int32.MinValue)
{
iTotalSecontds = System.Int32.MinValue;
}
else
{
iTotalSecontds = (int)dTotalSecontds;
}
if (iTotalSecontds > 0)
{
minTime = time2;
maxTime = time1;
}
else if (iTotalSecontds < 0)
{
minTime = time1;
maxTime = time2;
}
else
{
return time1;
}
int maxValue = iTotalSecontds;
if (iTotalSecontds <= System.Int32.MinValue)
maxValue = System.Int32.MinValue;
int i = random.Next(System.Math.Abs(maxValue));
return minTime.AddSeconds(i);
}
}
}
//测试
//MessageBox.Show(DateTimeHelper.GetRandomTime(DateTime.Parse("2008-09-27 00:00"),DateTime.Parse("2008-09-30 00:00")).ToString("yyyy-MM-dd hh:mm"))
--------------------编程问答--------------------
可否按照我上面的需求给个详细的示例,非常感谢. --------------------编程问答-------------------- 楼上回答的很详细 --------------------编程问答--------------------
请问你给多少钱? --------------------编程问答--------------------

using System;
namespace WindowsApplication2
{
public class DateTimeHelper
{
/**/
/// <summary>
/// 获取随机时间
/// <remarks>
/// 由于Random 以当前系统时间做为种值,所以当快速运行多次该方法所得到的结果可能相同,
/// 这时,您应该在外部初始化 Random 实例并调用 GetRandomTime(DateTime time1, DateTime time2, Random random)
/// </remarks>
/// </summary>
/// <param name="time1"></param>
/// <param name="time2"></param>
/// <returns></returns>
public static DateTime GetRandomTime(DateTime time1, DateTime time2)
{
Random random = new Random();
return GetRandomTime(time1, time2, random);
}
/**/
/// <summary>
/// 获取随机时间
/// </summary>
/// <param name="time1"></param>
/// <param name="time2"></param>
/// <param name="random"></param>
/// <returns></returns>
public static DateTime GetRandomTime(DateTime time1, DateTime time2, Random random)
{
DateTime minTime = new DateTime();
DateTime maxTime = new DateTime();
System.TimeSpan ts = new System.TimeSpan(time1.Ticks - time2.Ticks);
// 获取两个时间相隔的秒数
double dTotalSecontds = ts.TotalSeconds;
int iTotalSecontds = 0;
if (dTotalSecontds > System.Int32.MaxValue)
{
iTotalSecontds = System.Int32.MaxValue;
}
else if (dTotalSecontds < System.Int32.MinValue)
{
iTotalSecontds = System.Int32.MinValue;
}
else
{
iTotalSecontds = (int)dTotalSecontds;
}
if (iTotalSecontds > 0)
{
minTime = time2;
maxTime = time1;
}
else if (iTotalSecontds < 0)
{
minTime = time1;
maxTime = time2;
}
else
{
return time1;
}
int maxValue = iTotalSecontds;
if (iTotalSecontds <= System.Int32.MinValue)
maxValue = System.Int32.MinValue;
int i = random.Next(System.Math.Abs(maxValue));
return minTime.AddSeconds(i);
}
}
}
//测试
//MessageBox.Show(DateTimeHelper.GetRandomTime(DateTime.Parse("2008-09-27 00:00"),DateTime.Parse("2008-09-30 00:00")).ToString("yyyy-MM-dd hh:mm"))
可否按照我上面的需求给个详细的示例,非常感谢.
请问你给多少钱?
你问了我想问的了

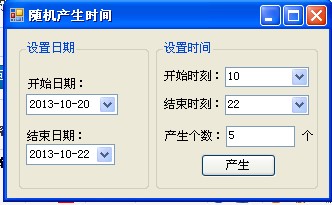
public void WriteMessage(string message)--------------------编程问答-------------------- 已经测试OK了,上面的代码共享了~
{
#region
if (!Directory.Exists(Application.StartupPath + "\\Result"))
Directory.CreateDirectory(Application.StartupPath + "\\Result");
string sPath = Application.StartupPath + "\\Result\\" + System.DateTime.Now.ToString("yyyy-MM-dd") + ".txt";
FileInfo fiInfo = new FileInfo(sPath);
StreamWriter swWriter = fiInfo.AppendText();
swWriter.WriteLine(message);
swWriter.Flush();
swWriter.Close();
#endregion
}
private void btnOK_Click(object sender, EventArgs e)
{
#region
bool isError = false;
int iHour = 0;
int iMinute = 0;
int iSecond = 0;
string sHour = string.Empty;
string sMinute = string.Empty;
string sSecond = string.Empty;
int iBeginTime = 0;
int iCount = 0;
int iEndTime = 0;
try
{
iBeginTime = int.Parse(this.beginTime.Text);
iEndTime = int.Parse(this.endTime.Text);
iCount = int.Parse(this.txtTimes.Text);
}
catch
{
isError = true;
MessageBox.Show("“设置时间”出错!请选择合理的时间间隔!", "警告", MessageBoxButtons.OK, MessageBoxIcon.Warning);
}
Random random = new Random();
string[] sArray = this.beginDate.Text.Split('-');
int iBeginYear = int.Parse(sArray[0]);
int iBeginMonth = int.Parse(sArray[1]);
int iBeginDay = int.Parse(sArray[2]);
DateTime dtTime1 = new DateTime(iBeginYear, iBeginMonth, iBeginDay);
sArray = this.endDate.Text.Split('-');
int iYear = int.Parse(sArray[0]);
int iMonth = int.Parse(sArray[1]);
int iDay = int.Parse(sArray[2]);
DateTime dtTime2 = new DateTime(iYear, iMonth, iDay);
TimeSpan tsSpan = dtTime2.Subtract(dtTime1);
int iMinusDay = tsSpan.Days;
string sBeginPart = string.Empty;
if (!isError)
{
for (int i = 0; i < iMinusDay + 1; i++)
{
sBeginPart = dtTime1.ToString("yyyy-MM-dd");
for (int j = 0; j < iCount; j++)
{
try
{
iHour = random.Next(iBeginTime, iEndTime);
}
catch
{
MessageBox.Show("“设置时间”出错!“开始时刻”不能大于“结束时刻”!", "警告", MessageBoxButtons.OK, MessageBoxIcon.Warning);
isError = true;
break;
}
if (iHour < 10)
sHour = "0" + iHour;
else sHour = "" + iHour;
iMinute = random.Next(0, 61);
if (iMinute < 10)
sMinute = "0" + iMinute;
else sMinute = "" + iMinute;
iSecond = random.Next(0, 61);
if (iSecond < 10)
sSecond = "0" + iSecond;
else sSecond = "" + iSecond;
WriteMessage(sBeginPart + " " + sHour + ":" + sMinute + ":" + sSecond);
MessageBox.Show(sBeginPart + " " + sHour + ":" + sMinute + ":" + sSecond);
}
WriteMessage(" ");
dtTime1 = dtTime1.AddDays(1);
}
if (!isError)
MessageBox.Show("数据产生完毕 ....");
}


补充:.NET技术 , C#