Java Swing 中的表格问题
先看代码:import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Component;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.util.EventObject;
import javax.swing.AbstractCellEditor;
import javax.swing.DefaultCellEditor;
import javax.swing.ImageIcon;
import javax.swing.JColorChooser;
import javax.swing.JComboBox;
import javax.swing.JDialog;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.UIManager;
import javax.swing.table.AbstractTableModel;
import javax.swing.table.TableCellEditor;
import javax.swing.table.TableCellRenderer;
import javax.swing.table.TableColumn;
import javax.swing.table.TableColumnModel;
import javax.swing.table.TableModel;
public class TableCellRenderTest {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
try {
UIManager
.setLookAndFeel("com.sun.java.swing.plaf.nimbus.NimbusLookAndFeel");
} catch (Throwable e) {
e.printStackTrace();
}
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
// TODO Auto-generated method stub
JFrame frame = new TableCellRenderFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
});
}
}
class TableCellRenderFrame extends JFrame {
private static final long serialVersionUID = 2017007572540121699L;
public TableCellRenderFrame() {
setTitle("TableCellRenderTest");
setSize(DEFAULT_WIDTH, DEFAULT_HEIGHT);
TableModel model = new PlanetTableModel();
JTable table = new JTable(model);
table.setRowSelectionAllowed(false);
// set up renderers and editors
table.setDefaultRenderer(Color.class, new ColorTableCellRenderer());
table.setDefaultEditor(Color.class, new ColorTableCellEditor());
JComboBox<Integer> moonCombo = new JComboBox<Integer>();
for (int i = 0; i <= 20; i++) {
moonCombo.addItem(i);
}
// 获取列视图
TableColumnModel columnModel = table.getColumnModel();
TableColumn moonColumn = columnModel
.getColumn(PlanetTableModel.MOONS_COLUMN);
moonColumn.setCellEditor(new DefaultCellEditor(moonCombo));
moonColumn.setHeaderRenderer(table.getDefaultRenderer(ImageIcon.class));
moonColumn.setHeaderValue(new ImageIcon("images/6.jpg"));
// show table
table.setRowHeight(100);
add(new JScrollPane(table), BorderLayout.CENTER);
}
private static final int DEFAULT_WIDTH = 600;
private static final int DEFAULT_HEIGHT = 400;
}
/*
* The planet table model specifies the value,rendering and editing properties
* for the planet data.
*/
class PlanetTableModel extends AbstractTableModel {
private static final long serialVersionUID = -6525995252603866044L;
// 返回列数
@Override
public int getColumnCount() {
// TODO Auto-generated method stub
return cells[0].length;
}
// 返回行数
@Override
public int getRowCount() {
// TODO Auto-generated method stub
return cells.length;
}
@Override
public Object getValueAt(int r, int c) {
// TODO Auto-generated method stub
return cells[r][c];
}
public String getColumnName(int c) {
return columnsName[c];
}
public Class<?> getColumnClass(int c) {
return cells[0][c].getClass();
}
public void setValueAt(Object obj, int r, int c) {
cells[r][c] = obj;
}
public boolean isCellEditable(int r, int c) {
return c == PLANET_COLUMN || c == MOONS_COLUMN || c == GASEOUS_COLUMN
|| c == COLOR_COLUMN;
}
public static final int PLANET_COLUMN = 0;
public static final int MOONS_COLUMN = 2;
public static final int GASEOUS_COLUMN = 3;
public static final int COLOR_COLUMN = 4;
private Object[][] cells = {
{ "Mercury", 2440.0, 0, false, Color.yellow,
new ImageIcon("images/7.jgp") },
{ "Venus", 6052.0, 0, false, Color.YELLOW,
new ImageIcon("images/8.jpg") },
{ "Earth", 6378.0, 0, false, Color.BLUE,
new ImageIcon("images/9.jpg") },
{ "Mars", 3397.0, 0, false, Color.RED,
new ImageIcon("images/10.jpg") },
{ "Jupiter", 71492.0, false, Color.ORANGE,
new ImageIcon("images/11.jpg") },
{ "Saturn", 60268.0, 0, false, Color.ORANGE,
new ImageIcon("images/12.jpg") },
{ "Uranus", 25559.0, 0, false, Color.BLUE,
new ImageIcon("images/13.jpg") },
{ "Neptune", 24766.0, 0, false, Color.BLUE,
new ImageIcon("images/14.jpg") },
{ "Pluto", 1137.0, 1, false, Color.BLACK,
new ImageIcon("images/15.jpg") }
};
private String[] columnsName = { "Planet", "Radius", "Moons", "Gaseous",
"Color", "Image" };
}
/*
* This renderer renders a color value as a panel with the given color.
*/
class ColorTableCellRenderer extends JPanel implements TableCellRenderer {
private static final long serialVersionUID = 851890999617120783L;
@Override
public Component getTableCellRendererComponent(JTable table, Object value,
boolean isSelected, boolean hasFocus, int row, int column) {
// TODO Auto-generated method stub
setBackground((Color) value);
if (hasFocus)
setBorder(UIManager.getBorder("Table.focusCellHighlightBorder"));
else
setBorder(null);
return this;
}
}
/*
* This editor pops up a color dialog to edit a cell value
*/
class ColorTableCellEditor extends AbstractCellEditor implements
TableCellEditor {
private static final long serialVersionUID = -5021717417421372502L;
// 构造器
public ColorTableCellEditor() {
panel = new JPanel();
// prepare color dialog
/*
* public static JDialog createDialog(Component c,String title,boolean
* modal,JColorChooser colorChooser,ActionLisntener
* okListener,ActionListener cancelListener);
*
* @param c 对话框的父组件
*
* @param title 对话框的标题
*
* @param modal 为true时,在关闭对话框之前,程序的剩余部分将一直处于非激活状态
*
* @param colorChooser 要置于对话框中的颜色选择器
*
* @param okListener 按下"OK"时调用的ActionListener
*
* @param calcelListener 按下"Cancel"时调用的ActionListener
*/
colorChooser = new JColorChooser();
colorDialog = JColorChooser.createDialog(null, "Planet Color", false,
colorChooser, new ActionListener() {
@Override
public void actionPerformed(ActionEvent event) {
// TODO Auto-generated method stub
stopCellEditing();
}
}, new ActionListener() {
@Override
public void actionPerformed(ActionEvent event) {
// TODO Auto-generated method stub
cancelCellEditing();
}
});
colorDialog.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent arg0) {
// TODO Auto-generated method stub
cancelCellEditing();
}
});
}
@Override
public Object getCellEditorValue() {
// TODO Auto-generated method stub
return colorChooser.getColor();
}
/*
* Component getTableCellEditorComponent(JTable table, Object value, boolean
* isSelected, int row, int column)
*/
@Override
public Component getTableCellEditorComponent(JTable table, Object value,
boolean isSelected, int row, int column) {
// TODO Auto-generated method stub
// this is where we get the current Color value,We store it in the
// dialog in case
// the user starts editing
colorChooser.setColor((Color) value);
return panel;
}
public boolean shouldSelectCell(EventObject anEvent) {
// start editing
colorChooser.setVisible(true);
// tell caller it is ok to select this cell
return true;
}
// 告知编辑器取消编辑并且不接受任何已部分编辑的值。
public void cancelCellEditing() {
// editing is canceled--hide dialog
colorDialog.setVisible(false);
super.cancelCellEditing();
}
// 告知编辑器停止编辑并接受任何已部分编辑的值作为编辑器的值。
// 如果编辑没有停止,则编辑器返回 false;这对已生效并且无法接受无效条目的编辑器很有用。
public boolean stopCellEditing() {
// editing is complete--hide dialog
colorDialog.setVisible(false);
super.stopCellEditing();
// tell caller it is ok to use color value
return true;
}
private JColorChooser colorChooser;
private JDialog colorDialog;
private JPanel panel;
}
运行截图:
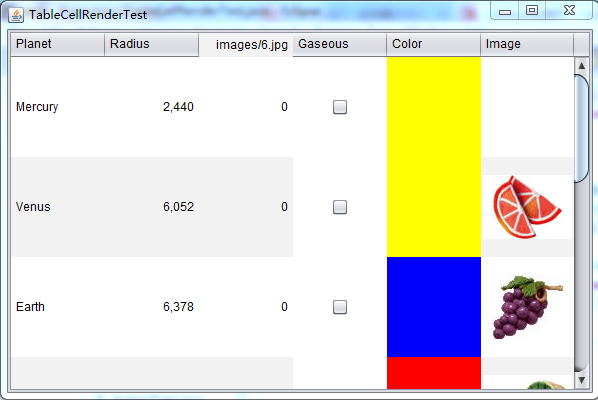
问题:
当下拉滚动条时出现了错误,看Console截图:

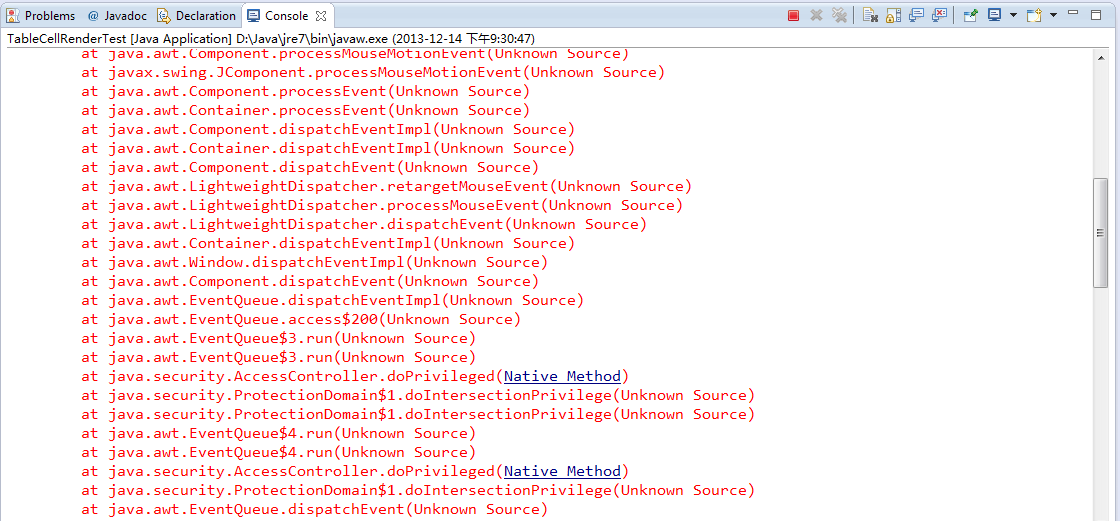
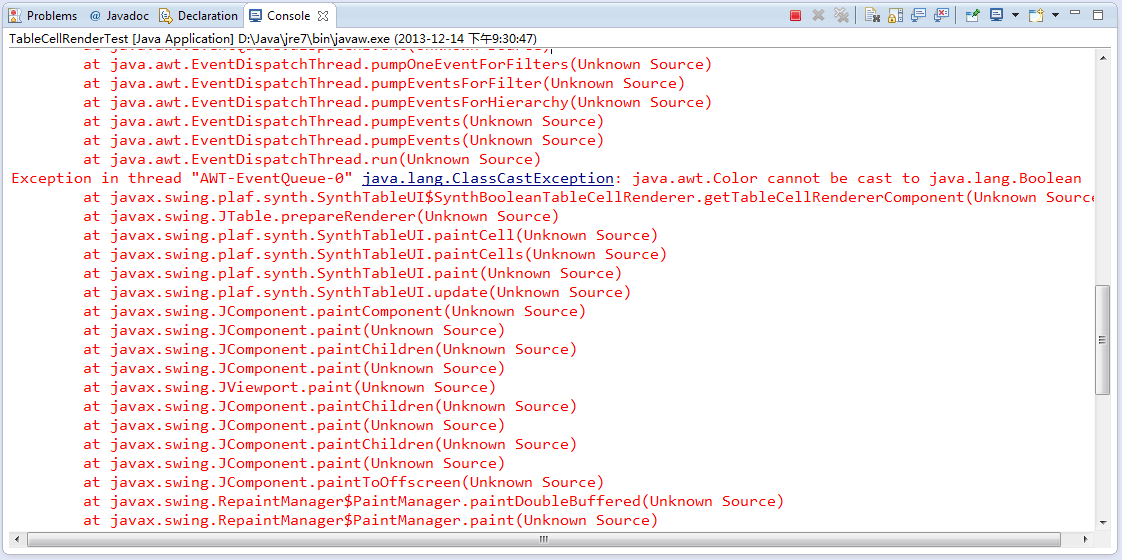
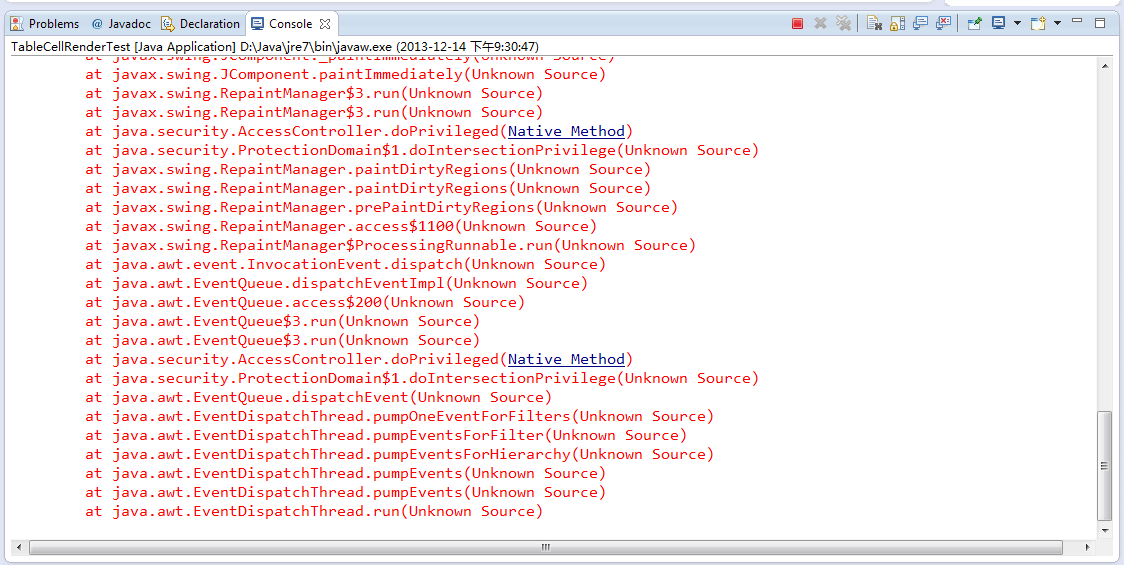
--------------------编程问答-------------------- 除
补充:Java , Java SE