C#局域视频,资源源捕获源固定
因为我的电脑有一个类似于电视的资源源,还有2个摄像头。每次测试我这个局域视频功能的时候,总会出现一个对话框“资源源->捕获源”,然后让我选择这3个资源的哪一个。有没有什么办法让我直接固定到我的一个摄像头上,以后直接使用呢?
新手,一下是Video类 和 开启视频功能的代码
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Runtime.InteropServices;
using System.Drawing.Imaging;
using System.Drawing;
namespace video
{
public class Video
{
//5个传入参数
private IntPtr myHand;
private int myWidth;
private short myHeight;
private int myLeft;
private int myTop;
//调用avicap32.dll
public struct videohdr_tag
{
public byte[] lpData;
public int dwBufferLength;
public int dwBytesUsed;
public int dwTimeCaptured;
public int dwUser;
public int dwFlags;
public int[] dwReserved;
}
[DllImport("avicap32.dll", CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern int capCreateCaptureWindowA([MarshalAs(UnmanagedType.VBByRefStr)] ref string lpszWindowName, int dwStyle, int x, int y, int nWidth, short nHeight, int hWndParent, int nID);
[DllImport("avicap32.dll", CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern bool capGetDriverDescriptionA(short wDriver, [MarshalAs(UnmanagedType.VBByRefStr)] ref string lpszName, int cbName, [MarshalAs(UnmanagedType.VBByRefStr)] ref string lpszVer, int cbVer);
[DllImport("user32", CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern bool DestroyWindow(int hndw);
[DllImport("user32", EntryPoint = "SendMessageA", CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern int SendMessage(int hwnd, int wMsg, int wParam, [MarshalAs(UnmanagedType.AsAny)] object lParam);
[DllImport("user32", CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern int SetWindowPos(int hwnd, int hWndInsertAfter, int x, int y, int cx, int cy, int wFlags);
[DllImport("vfw32.dll")]
public static extern string capVideoStreamCallback(int hwnd, videohdr_tag videohdr_tag);
[DllImport("vicap32.dll", CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern bool capSetCallbackOnFrame(int hwnd, string s);
//自定义参数:
private int hHwnd;
//构造函数
public Video(IntPtr myPtr, int left, int top, int width, short height)
{
myHand = myPtr;
myLeft = left;
myTop = top;
myWidth = width;
myHeight = height;
//
// TODO: 在此处添加构造函数逻辑
//
}
//打开视频:
public void opVideo()
{
int intDevice = 0;
string refDevice = intDevice.ToString();
//MessageBox.Show("1");
hHwnd = capCreateCaptureWindowA(ref refDevice, 1342177280, 0, 0, 1024, 768, myHand.ToInt32(), 0);
//MessageBox.Show("2");
if (SendMessage(hHwnd, 0x40a, intDevice, 0) > 0)
{
//MessageBox.Show("9");
SendMessage(this.hHwnd, 0x435, -1, 0);//设置预览规模
//MessageBox.Show("3");
SendMessage(this.hHwnd, 0x434, 100, 0);//设置以毫秒计算预览率
//MessageBox.Show("4");
SendMessage(this.hHwnd, 0x432, -1, 0);//设置预览,开始预览从相机的图像/capCreateCaptureWindowAppppppppppppppppppppl
//MessageBox.Show("5");
SetWindowPos(this.hHwnd, 1, 0, 0, myWidth, Convert.ToInt32(myHeight), 6);
//MessageBox.Show("6");
}
else
{
DestroyWindow(this.hHwnd);
}
}
//停止视频
public void CloVideo()
{
SendMessage(this.hHwnd, 0x40b, 0, 0);
DestroyWindow(this.hHwnd);
}
//捕获视频
public Image CatchVideo()
{
SendMessage(this.hHwnd, 0x41e, 0, 0);
IDataObject obj1 = Clipboard.GetDataObject();
//MessageBox.Show(this.hHwnd.ToString());
Image getIma = null;
if (obj1.GetDataPresent(typeof(Bitmap)))
{
Image image1 = (Image)obj1.GetData(typeof(Bitmap));
getIma = image1;
}
return getIma;
}
public void GrabImage(string path)
{
try
{
IntPtr hBmp = Marshal.StringToHGlobalAnsi(path);
SendMessage(hHwnd, 0x400 + 25, 0, hBmp.ToInt64());
}
catch
{
}
}
}
}
--------------------编程问答-------------------- 如果你的系统里装有多个捕获设备, 你可以在发送WM_CAP_DRIVER_CONNECT消息时用wParam参数指定使用哪一个, 此参数是登记在SYSTEM.INI文件的[drivers]一节里的列表中的某一项, 0为第一个.
public Form1(String IP)
{
InitializeComponent();
Video = new Video(Image_small.Handle, this.Image_small.Left, this.Image_small.Top, this.Image_small.Width, (short)this.Image_small.Height);
IP_udp = IP;
}
private void Form1_Load(object sender, EventArgs e)
{
threadVideo = new Thread(new ThreadStart(this.StartListenVideo));
threadVideo.IsBackground = true;
threadVideo.Start();
Video.opVideo();
timer.Enabled = true;
}
public void VideoInvoke()
{
this.Image_big.Image = this.ReceivedImage;
}
public void StartListenVideo()
{
udpclient = new UdpClient(12000);
IPEndPoint ipendpoint = new IPEndPoint(ip, 12000);
while (true)
{
try
{
byte[] bytes = udpclient.Receive(ref ipendpoint);
MemoryStream ms = new MemoryStream(bytes);
ReceivedImage = Image.FromStream(ms);
this.Image_big.Invoke(new MyDelegate(this.VideoInvoke));
}
catch (Exception ex)
{
}
}
}
private void timer_Tick(object sender, EventArgs e)
{
VideoImage = Video.CatchVideo();
imgSender.SendImage(IP_udp, 13000, VideoImage);
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
udpclient.Close();
threadVideo.Abort();
}
SendMessage(hHwnd, WM_CAP_DRIVER_CONNECT, 0, 0); --------------------编程问答--------------------
还是不懂,没能成功,
跟capGetDriverDescriptionA , capCreateCaptureWindowA没有关系吗? --------------------编程问答-------------------- 你本地是不是有多个摄像头设备! --------------------编程问答--------------------
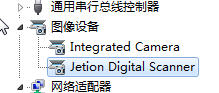
本地2个摄像头,默认打开第1个摄像头,如何选第2个?求教大大!
SendMessage(hHwnd, WM_CAP_DRIVER_CONNECT, intDevice , 0);
使用第一个摄像头时,intDevice=0;使用第2个时,intDevice=1不行;
另:system.ini里未找到drivers节
/// <summary>
///开始载入视频
/// </summary>
public bool OpenCapture(Panel pnlVideo, int intDevice) //开始载入视频
{
bool videostart = false;
int intWidth = pnlVideo.Width;
int intHeight = pnlVideo.Height;
//第1个摄像头================
string refDevice = intDevice.ToString();
//创建视频窗口并得到句柄
hHwnd = Camera.capCreateCaptureWindowA(ref refDevice, 1342177280, 0, 0, 640, 480, pnlVideo.Handle.ToInt32(), 0);
SendMessage(hHwnd, WM_CAP_DRIVER_DISCONNECT, intDevice, 0);
SendMessage(hHwnd, WM_CAP_DRIVER_CONNECT, intDevice , 0);
SendMessage(hHwnd, WM_CAP_SET_PREVIEW,true , 0);
if (SendMessage(hHwnd, 0x40a, intDevice, 0) > 0)
{
SendMessage(hHwnd, 0x435, -1, 0);
SendMessage(hHwnd, 0x434, 0x01, 0);
SendMessage(hHwnd, 0x432, -1, 0);
SetWindowPos(hHwnd, 1, 0, 0, intWidth, intHeight, 6);
videostart = true;
}
else
{
Camera.DestroyWindow(hHwnd);
videostart = false;
MessageBox.Show("加载视频失败,请检查是否有安装设备!", "拍照");
}
return videostart;
}
补充:.NET技术 , C#