HttpWebRequest登陆开心网
最近学HttpWebRequest和HttpWebResponse的应用,可以模拟登陆一些简单的网站了。不过开心网我看不懂请大家帮我分析一下行么?为什么一开始有两个post,我改用代码怎么模拟?写个思路就可以。
一般性我知道只要post给他我的用户名,密码然后返回一个cookie,然后带着这个cookie get下一个登录后的页面就能拿到数据了。可是开心网怎么弄。我用firefox的firebug看了老半天没看懂。 --------------------编程问答-------------------- 你去下一个开心网的外挂 看看就知道了。比如说停车外挂 --------------------编程问答-------------------- 没有源码的吧,而且开心网一直在更新,有哪个能用的么。。 --------------------编程问答-------------------- mark,明天帮你看。 --------------------编程问答--------------------
--------------------编程问答-------------------- //---------------登录
#region 同步通过POST方式发送数据
/// <summary>
/// 通过POST方式发送数据
/// </summary>
/// <param name="Url">url</param>
/// <param name="postDataStr">Post数据</param>
/// <param name="cookie">Cookie容器</param>
/// <returns></returns>
public static string SendDataByPost(string Url, string postDataStr, ref CookieContainer cookie)
{
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(Url);
if (cookie.Count == 0)
{
request.CookieContainer = new CookieContainer();
cookie = request.CookieContainer;
}
else
{
request.CookieContainer = cookie;
}
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
request.ContentLength = postDataStr.Length;
Stream myRequestStream = request.GetRequestStream();
StreamWriter myStreamWriter = new StreamWriter(myRequestStream, Encoding.GetEncoding("gb2312"));
myStreamWriter.Write(postDataStr);
myStreamWriter.Close();
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
Stream myResponseStream = response.GetResponseStream();
StreamReader myStreamReader = new StreamReader(myResponseStream, Encoding.GetEncoding("utf-8"));
string retString = myStreamReader.ReadToEnd();
myStreamReader.Close();
myResponseStream.Close();
return retString;
}
#endregion
#region 同步通过GET方式发送数据
/// <summary>
/// 通过GET方式发送数据
/// </summary>
/// <param name="Url">url</param>
/// <param name="postDataStr">GET数据</param>
/// <param name="cookie">GET容器</param>
/// <returns></returns>
public static string SendDataByGET(string Url, string postDataStr, ref CookieContainer cookie)
{
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(Url + (postDataStr == "" ? "" : "?") + postDataStr);
if (cookie.Count == 0)
{
request.CookieContainer = new CookieContainer();
cookie = request.CookieContainer;
}
else
{
request.CookieContainer = cookie;
}
request.Method = "GET";
request.ContentType = "application/x-www-form-urlencoded";
request.Referer = "www";
// "https://passport.csdn.net/account/loginbox?callback=logined&hidethird=1&from=http%3a%2f%2fbbs.csdn.net%2f";
//request.Headers
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
Stream myResponseStream = response.GetResponseStream();
StreamReader myStreamReader = new StreamReader(myResponseStream, Encoding.GetEncoding("utf-8"));
string retString = myStreamReader.ReadToEnd();
myStreamReader.Close();
myResponseStream.Close();
return retString;
}
#endregion
string url = "https://security.kaixin001.com/login/login_auth.php";
string postData = "email=你的帐号,我这边测试用的是我的email&password=123456&rcode=";
string receBody = SendDataByPost(url, postData, ref cookieContainer);
//------------------------用返回的这个cookieContainer去GET访问其他页面。
string sdsds = SendDataByGET(url, "", ref cookieContainer);
--------------------编程问答-------------------- 标记下。
下午过来学习 --------------------编程问答--------------------
你测试成功了吗,成功取得登陆后的html了吗? --------------------编程问答-------------------- 我好像还是取不到。你这个对吗。。 --------------------编程问答--------------------
public class HttpHelper
{
public string contentType = "application/x-www-form-urlencoded";
public string accept = "text/html, application/xhtml+xml, */*";
public string userAgent = "Mozilla/5.0 (compatible; MSIE 10.0; Windows NT 6.2; WOW64; Trident/6.0)";
public string referer = string.Empty;
[DllImport("wininet.dll", CharSet = CharSet.Auto, SetLastError = true)]
public static extern bool InternetSetCookie(string lpszUrl, string lpszCookieName, string lpszCookieData);
/// <summary>
/// 获取流数据
/// </summary>
/// <param name="url"></param>
/// <returns></returns>
public Stream GetStream(string url, CookieContainer cookies = null)
{
cookies = cookies ?? new CookieContainer();
HttpWebRequest httpWebRequest = null;
HttpWebResponse httpWebResponse = null;
try
{
httpWebRequest = (HttpWebRequest)HttpWebRequest.Create(url);
httpWebRequest.CookieContainer = cookies;
httpWebRequest.ContentType = contentType;
httpWebRequest.Referer = referer;
httpWebRequest.Accept = accept;
httpWebRequest.UserAgent = userAgent;
httpWebRequest.Method = "GET";
httpWebRequest.ServicePoint.ConnectionLimit = int.MaxValue;
//WebProxy proxy = new WebProxy("web-proxy.houston.hp.com", 8080);
//httpWebRequest.Proxy = proxy;
httpWebResponse = (HttpWebResponse)httpWebRequest.GetResponse();
Stream responseStream = httpWebResponse.GetResponseStream();
return responseStream;
}
catch (Exception)
{
return null;
}
}
/// <summary>
/// 获取HTML
/// </summary>
/// <param name="url"></param>
/// <param name="cookieContainer"></param>
/// <param name="method">GET or POST</param>
/// <param name="postData">like "username=admin&password=123"</param>
/// <returns></returns>
public string GetHtml(string url, CookieContainer cookies = null, string method = "GET", string postData = "")
{
cookies = cookies ?? new CookieContainer();
HttpWebRequest httpWebRequest = null;
HttpWebResponse httpWebResponse = null;
try
{
httpWebRequest = (HttpWebRequest)HttpWebRequest.Create(url);
httpWebRequest.CookieContainer = cookies;
httpWebRequest.ContentType = contentType;
httpWebRequest.Referer = referer;
httpWebRequest.Accept = accept;
httpWebRequest.UserAgent = userAgent;
httpWebRequest.Method = method;
httpWebRequest.ServicePoint.ConnectionLimit = int.MaxValue;
httpWebRequest.AllowAutoRedirect = true;
//WebProxy proxy = new WebProxy("web-proxy.houston.hp.com", 8080);
//httpWebRequest.Proxy = proxy;
if (method.ToUpper() == "POST" && postData.Length > 0)
{
byte[] byteRequest = Encoding.UTF8.GetBytes(postData);
Stream stream = httpWebRequest.GetRequestStream();
stream.Write(byteRequest, 0, byteRequest.Length);
stream.Close();
}
httpWebResponse = (HttpWebResponse)httpWebRequest.GetResponse();
Stream responseStream = httpWebResponse.GetResponseStream();
StreamReader streamReader = new StreamReader(responseStream, Encoding.UTF8);
string html = streamReader.ReadToEnd();
streamReader.Close();
responseStream.Close();
httpWebRequest.Abort();
httpWebResponse.Close();
return html;
}
catch (Exception ex)
{
return ex.Message;
}
}
}
发一个我的给你。
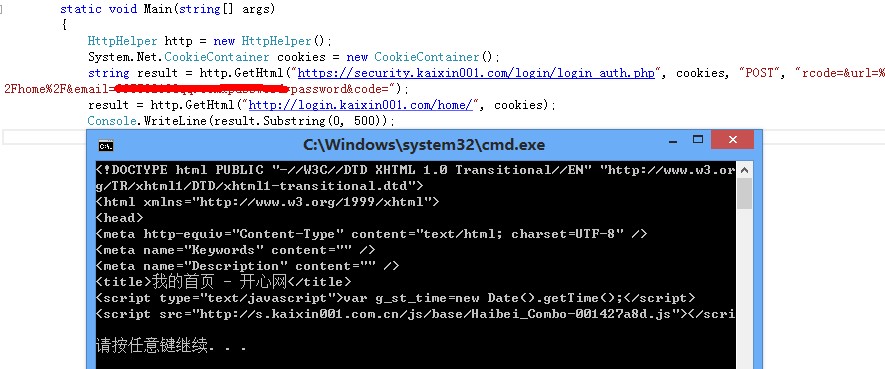
--------------------编程问答-------------------- 一开始post 的 http://www.kaixin001.com/interface/statLog2.php 没什么用的吗?
static void Main(string[] args)
{
HttpHelper http = new HttpHelper();
System.Net.CookieContainer cookies = new CookieContainer();
string result = http.GetHtml("https://security.kaixin001.com/login/login_auth.php", cookies, "POST", "rcode=&url=%2Fhome%2F&email=123456@qq.com&qazwsx=password&code=");
result = http.GetHtml("http://login.kaixin001.com/home/", cookies);
Console.WriteLine(result.Substring(0, 500));
}
补充:.NET技术 , C#