求递归算法
求解:1,1,2,3。。。。。m求第30位数的和,用递归做(C#语言)--------------------编程问答--------------------
public static int foo(int n)--------------------编程问答--------------------
{
if (n <= 2)
{
return 1;
}
return foo(n - 1) + foo(n - 2);
}
+++ --------------------编程问答--------------------
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace ConsoleApplication1
{
class Program
{
public static int foo(int n)
{
if (n <= 2)
{
return 1;
}
return foo(n - 1) + foo(n - 2);
}
static void Main(string[] args)
{
int sum = 0;
for (int i = 1; i <= 30; i++)
{
sum += foo(i);
}
Console.WriteLine(sum);
}
}
}
2178308 --------------------编程问答-------------------- 再给你个恶搞老师的版本:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace ConsoleApplication1
{
class Program
{
static IEnumerable<int> Fibonacci(int Max)
{
if (Max == 1)
{
yield return 1;
}
if (Max == 2)
{
yield return 1;
yield return 1;
}
if (Max > 2)
{
foreach (var i in Fibonacci(Max - 1)) yield return i;
yield return Fibonacci(Max - 1).Skip(Max - 3).Sum();
}
}
static void Main(string[] args)
{
Console.WriteLine(Fibonacci(30).Sum());
}
}
}
这个程序绝对正确,只是很慢。 --------------------编程问答--------------------
这个好! --------------------编程问答--------------------
++ --------------------编程问答-------------------- int doit(n)
{
return (n<=2?1:doit(--n)+doit(--1)
} --------------------编程问答-------------------- +++++ --------------------编程问答-------------------- 发错了~~~~ --------------------编程问答-------------------- 菲波拉契数列,规律是第一个和第二个数都为1,从第三个数开始,每个数是其前面两个数的和,很好做的
--------------------编程问答-------------------- 不知道你是要求数列的前n个元素的和,还是求第n个元素的值,上面的代码求的是第n个值。
/// <summary>
/// 计算斐波那契数列的第n个元素的值
/// </summary>
/// <param name="n">斐波那契数列的元素下标</param>
/// <returns></returns>
public static int Fab(int n)
{
// 返回值
int result = 0;
// 递归过程
if (n > 2)
{
result = Fab(n - 1) + Fab(n - 2);
}
if (n == 2)
{
result = 1;
}
if (n == 1)
{
result = 1;
}
return result;
}
下面的是求数列的前n个元素的和:
static void Main(string[] args)
{
int n = 4;
int sum = 0;
for (int i = 1; i < 5; i++)
{
sum = sum + Fibonacci.Fab(i);
}
int f = Fibonacci.Fab(n);
Console.WriteLine("前{0}项Fabonacci数列的和是{1};\r\n第{2}项Fabonacci数列的的值是{3}", n,sum,n,f);
Console.ReadLine();
}
结果如下
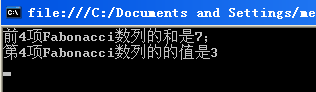

+++ --------------------编程问答--------------------
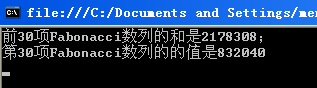
{
if(input<=0)
return 0;
else if(input>0 && input <=2)
return 1;
else
return Find(input-1)+Find(input-2);
}
调用函数
Find(30); --------------------编程问答-------------------- 确实有够慢,-O(∩_∩)O~-
--------------------编程问答-------------------- 要理解递归,首先要理解递归.... --------------------编程问答-------------------- 楼上答案有了。
--------------------编程问答--------------------
++ --------------------编程问答-------------------- 学习了,还没领悟
补充:.NET技术 , C#