Windows Phone 7 程序等待页面的处理
程序启动通常会有一个等待的过程,在这个过程中可以通过使用Popup控件配合BackgroundWorker类启动后台线程来实现。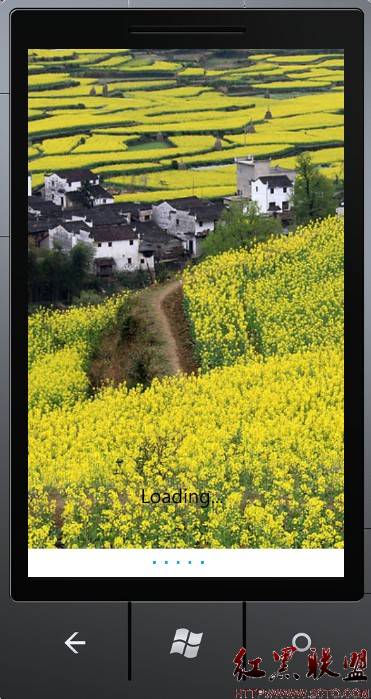
控件的代码
PopupSplash.xaml
<UserControl x:Class="ProgressSplashScreen.PopupSplash"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
FontFamily="{StaticResource PhoneFontFamilyNormal}"
FontSize="{StaticResource PhoneFontSizeNormal}"
Foreground="{StaticResource PhoneForegroundBrush}"
d:DesignHeight="800" d:DesignWidth="480">
<Grid x:Name="LayoutRoot" Background="White" Width="480" Height="800">
<ProgressBar HorizontalAlignment="Left" Margin="0,755,0,0" Name="progressBar1" Width="480" Background="DarkRed"/>
<Image Height="757" HorizontalAlignment="Left" Name="image1" Stretch="Fill" VerticalAlignment="Top" Width="480" Source="/ProgressSplashScreen;component/wuyuan.png"/>
<TextBlock HorizontalAlignment="Left" Margin="171,656,0,97" Name="textBlock1" Text="Loading..." Width="208" Foreground="Black" FontSize="30"/>
</Grid>
</UserControl>
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace ProgressSplashScreen
{
publicpartialclass PopupSplash : UserControl
{
public PopupSplash()
{
InitializeComponent();
this.progressBar1.IsIndeterminate =true;//指示进度条是使用重复模式报告一般进度
}
}
}
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using Microsoft.Phone.Controls;
using System.Windows.Controls.Primitives;
using System.ComponentModel;
using System.Threading;
namespace ProgressSplashScreen
{
publicpartialclass MainPage : PhoneApplicationPage
{
private Popup popup;
private BackgroundWorker backroungWorker;
public MainPage()
{
InitializeComponent();
ShowPopup();
}
privatevoid ShowPopup()
{
this.popup =new Popup();
this.popup.Child =new PopupSplash();//设置 Popup 控件的内容,把自定义的PopupSplash控件填充到popup控件上
this.popup.IsOpen =true;
StartLoadingData();//开始加载数据
}
privatevoid StartLoadingData()
{
//使用BackgroundWorker在单独的线程上执行操作
backroungWorker =new BackgroundWorker();
//调用 RunWorkerAsync后台操作时引发此事件,即后台要处理的事情写在这个事件里面
backroungWorker.DoWork +=new DoWorkEventHandler(backroungWorker_DoWork);
//当后台操作完成事件
backroungWorker.RunWorkerCompleted +=new RunWorkerCompletedEventHandler(backroungWorker_RunWorkerCompleted);
//开始执行后台操作
backroungWorker.RunWorkerAsync();
}
//后台操作完成
void backroungWorker_RunWorkerCompleted(object sender, RunWorkerCompletedEventArgs e)
{
this.Dispatcher.BeginInvoke(() =>
{
this.popup.IsOpen =false;//关闭popup 注意要使用Dispatcher.BeginInvoke开跟UI通讯
}
);
}
//后台操作处理
void backroungWorker_DoWork(object sender, DoWorkEventArgs e)
{
// 程序初始化处理 这里只是模拟了一下
Thread.Sleep(7000);
}
}
}
System.Windows.Controls.Primitives.Popup 类
xaml语法
<Popup>
Child
</Popup>
Popup 控件可在单独窗口中相对于屏幕上的元素或点显示内容。当 Popup 可见时,IsOpen 属性设置为 true。
Popup.Child 属性
内容模型:Child 属性是 Popup 控件的唯一内容属性。一个 Popup 只能有一个 UIElement 作为子级,但该子级可以包含复杂的嵌入内容。例如,该子级可以是包含 Image、文本和其他类型控件的 StackPanel。 当将内容添加到 Popup 控件时,Popup 控件会成为该内容的逻辑父级。同样,Popup 内容将被视为 Popup 的逻辑子级。不会将子内容添加到包含 Popup 控件的可视化树中。但当 IsOpen 设置为 true 时,子内容将呈现在具有自身可视化树的单独的窗口中。
System.ComponentModel. BackgroundWorker 类
BackgroundWorker 类允许您在单独的专用线程上运行操作。耗时的操作(如下载和数据库事务)在长时间运行时可能会导致用户界面 (UI) 似乎处于停止响应状态。如果您需要能进行响应的用户界面,而且面临与这类操作相关的长时间延迟,则可以使用 BackgroundWorker 类方便地解决问题。
若要在后台执行耗时的操作,请创建一个 BackgroundWorker,侦听那些报告操作进度并在操作完成时发出信号的事件。若要设置后台操作,请为 DoWork 事件添加一个事件处理程序。在此事件处理程序中调用耗时的操作。若要启动该操作,请调用 RunWorkerAsync。若要收到进度更新通知,请对 ProgressChanged 事件进行处理。若要在操作完成时收到通知,请对 RunWorkerCompleted 事件进行处理。
注意
您必须非常小心,确保在 DoWork 事件处理程序中不操作任何用户界面对象。而应该通过 ProgressChanged 和 RunWorkerCompleted 事件与用户界面进行通信。
BackgroundWorker 事件不跨 AppDomain 边界进行封送处理。请不要使用 BackgroundWorker 组件在多个 AppDomain 中执行多线程操作。
如果后台操作需要参数,请在调用 RunWorkerAsync 时给出参数。在 DoWork 事件处理程序内部,可以从 DoWorkEventArgs.Argument 属性中提取该参数。
摘自 逍遥谷
补充:移动开发 , Windows Phone ,