写了个类,在Console里面高仿win7复制文件提示覆盖选项
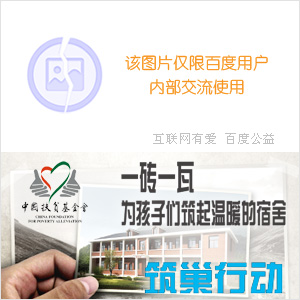
public static class CopyAndMove
{
public static void CopyTo(this string source, string target)
{
// 判断源路径是否为目录,若不是目录,再判断是否为文件。
if (!Directory.Exists(source))
{
// 若为文件,则将其复制到目标文件夹。
if (File.Exists(source))
CopyFile(source, target);
//否则,打印错误信息。
else
Console.WriteLine("The specified directory or file does not exist.");
Console.WriteLine("Press any key to exit...");
Console.ReadKey();
return;
}
CopyTree(source,target);
Console.WriteLine("Press any key to exit...");
Console.ReadKey();
}
public static void MoveTo(this string source, string target)
{
CopyTo(source, target);
if (Directory.Exists(source) || File.Exists(source))
if (Directory.Exists(target))
{
try
{
Directory.Delete(source, true);
}
catch
{
File.Delete(source);
}
}
}
static void CopyTree(string source,string target)
{
if (!ExistOrCreate(target))
return;
DirectoryInfo sourceFolder = new DirectoryInfo(source);
string targetDirectory = target.Trim('\\') + "\\" + sourceFolder.Name;
// 如果目标目录中存在同名文件夹,问是否融合。
if (Directory.Exists(targetDirectory))
{
Console.WriteLine("This destination already contains a folder named '{0}'.",
sourceFolder.Name);
Console.WriteLine("If any files have the same names, you will be asked " +
"if you want to replace those files.");
Console.WriteLine("Do you still want to merge this folder");
Console.WriteLine(" {0}", sourceFolder.Name);
Console.WriteLine(" Date created: {0}", sourceFolder.CreationTime.ToString());
Console.WriteLine("with this one?");
Console.WriteLine(" {0}", sourceFolder.Name);
Console.WriteLine(" Date created: {0}", (new DirectoryInfo(targetDirectory)).CreationTime.ToString());
Console.Write("Press (Y/y) to confirm, or any key else to abolish:");
string ans = Console.ReadLine().Trim().ToLower();
if (ans != "y")
{
Console.WriteLine("Merge abolished.\nPress any key to continue...");
Console.ReadKey();
return;
}
}
//若没有,则创建
else
Directory.CreateDirectory(targetDirectory);
// 计算同名文件个数
DirectoryInfo targetFolder = new DirectoryInfo(targetDirectory);
int count=0;
foreach(FileInfo file in sourceFolder.GetFiles())
foreach(FileInfo file2 in targetFolder.GetFiles())
if (file.Name.ToLower() == file2.Name.ToLower())
{
count++;
break;
}
bool ask = true, skip=false;
string operate = "";
//先拷贝文件
foreach (FileInfo file in sourceFolder.GetFiles())
{
if (skip)
break;
CopyFile(file.FullName, targetDirectory, ref ask, ref skip, ref operate, ref count);
}
//再拷目录
foreach (DirectoryInfo directory in sourceFolder.GetDirectories())
CopyTree(directory.FullName, targetDirectory);
}
static void CopyFile(string source, string target,ref bool ask,ref bool skip, ref string operate,ref int count)
{
if (!ExistOrCreate(target))
return;
FileInfo sourceFile=new FileInfo(source);
string targetFile = target.Trim('\\') + "\\" + sourceFile.Name;
if (File.Exists(targetFile))
{
count--;
string destName="";
string suffix=null;
if (sourceFile.Name.Contains('.'))
{
suffix = "." + sourceFile.Name.Split('.')[sourceFile.Name.Split('.').Length - 1];
foreach (string str in sourceFile.Name.Split('.').Take(sourceFile.Name.Split('.').Length - 1))
destName += str + ".";
destName = destName.Trim('.');
}
else
{
destName=sourceFile.Name;
suffix="";
}
int i=2;
targetFile=string.Format("{0}\\{1}(2){2}",target.Trim('\\'),destName,suffix);
for(;File.Exists(targetFile);)
targetFile=string.Format("{0}\\{1}({3}){2}",target.Trim('\\'),destName,suffix,++i);
if (ask)
{
Console.WriteLine("There is already a file with the same name in this location.");
Console.WriteLine("Press 1 to Copy and Replace;");
Console.WriteLine("Press 2 not to copy;");
Console.WriteLine("Press 3 to copy and keep both files, and the file you are " +
"copying will be renamed \"{0}({1}){2}\"", destName, i, suffix);
Console.WriteLine("Press 4 to skip this directory.");
Console.WriteLine("Press any other key to cancel.");
string ans = Console.ReadLine();
operate = ans;
if (count > 0 && operate!="4")
{
Console.WriteLine("Press Y/y to do this for the next {0} conflict{1},or any other key if not.",
count,count>1?"s":"");
ans = Console.ReadLine().Trim().ToUpper();
if (ans == "Y")
ask = false;
}
}
switch (operate)
{
case "1":
targetFile=target.Trim('\\') + "\\" + sourceFile.Name;
break;
case "3":
break;
case "4":
skip = true;
return;
default:
Console.WriteLine("Copy canceled.");
return;
}
}
File.Copy(sourceFile.FullName, targetFile, true);
Console.WriteLine("{0} copied to {1}", sourceFile.FullName, targetFile);
}
// 未完待续。。
--------------------编程问答-------------------- --------------------编程问答-------------------- 图片挂了 --------------------编程问答-------------------- 支持一下 --------------------编程问答-------------------- --------------------编程问答--------------------
static void CopyFile(string source, string target)
{
if (!ExistOrCreate(target))
return;
FileInfo sourceFile = new FileInfo(source);
string targetFile = target.Trim('\\') + "\\" + sourceFile.Name;
if (File.Exists(targetFile))
{
string destName = "";
string suffix = null;
if (sourceFile.Name.Contains('.'))
{
suffix = "." + sourceFile.Name.Split('.')[sourceFile.Name.Split('.').Length - 1];
foreach (string str in sourceFile.Name.Split('.').Take(sourceFile.Name.Split('.').Length - 1))
destName += str + ".";
destName = destName.Trim('.');
}
else
{
destName = sourceFile.Name;
suffix = "";
}
int i = 2;
targetFile = string.Format("{0}\\{1}(2){2}", target.Trim('\\'), destName, suffix);
for (; File.Exists(targetFile); )
targetFile = string.Format("{0}\\{1}({3}){2}", target.Trim('\\'), destName, suffix, ++i);
Console.WriteLine("There is already a file with the same name in this location.");
Console.WriteLine("Press 1 to Copy and Replace;");
Console.WriteLine("Press 2 not to copy;");
Console.WriteLine("Press 3 to copy and keep both files, and the file you are " +
"copying will be renamed \"{0}({1}){2}\"", destName, i, suffix);
Console.WriteLine("Press any other key to cancel.");
string ans = Console.ReadLine();
switch (ans)
{
case "1":
targetFile = target.Trim('\\') + "\\" + sourceFile.Name;
break;
case "3":
break;
default:
Console.WriteLine("Copy canceled.");
return;
}
}
File.Copy(sourceFile.FullName, targetFile, true);
Console.WriteLine("{0} copied to {1}", sourceFile.FullName, targetFile);
}
static bool ExistOrCreate(string target)
{
// check whether the destination directory exists. Create one if not.
if (!Directory.Exists(target))
{
Console.WriteLine("Target directory not found.");
try
{
Console.WriteLine("Try to create one...");
Directory.CreateDirectory(target);
Console.WriteLine("Destination folder created.");
}
// If creation failed, print error message and quit.
catch (Exception e)
{
Console.WriteLine("Creation failes.\n"+e.Message+"\nPress any key to continue...");
Console.ReadKey();
return false;
}
}
return true;
}
}
补充:.NET技术 , C#