编了个java小程序,有问题哦!
编了一个java小程序,画一条魚,魚嘴里不断吐泡泡。运行不了,不知道哪里出错了。有空帮我看看啦。
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
class Fish extends Frame implements ActionListener {
Button start = new Button("吐泡");
Fish fish[] = new Fish[5];
public Fish() {
super("Fish");
add(start);
start.addActionListener(this);
setSize(500,500);
setVisible(true);
setLayout(new FlowLayout());
validate();
addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent we)
{System.exit(0);}});
public void actionPerformed(ActionEvent ae) {
for(int i=0;i<fish.length;i++) {
//定义泡泡速度和坐标.
int ySpeed = 5;
int x = 108;
int y = 250;
//实例化线程.
fish[i] = new Fish(x,y,ySpeed);
fish[i].start();
}
}
public void paint(Graphics g2) {
for(int i=0;i<fish.length;i++)
if(fish[i] != null)
fish[i].draw(g2);
}
}
//画鱼.
public void paint(Graphics g) {
g.drawLine(110,230,250,110);
g.drawLine(110,270,250,390);
g.drawLine(110,230,130,250);
g.drawLine(110,270,130,250);
g.drawLine(250,230,300,200);
g.drawLine(250,270,300,300);
g.drawLine(300,200,300,300);
g.drawLine(250,110,250,390);
g.setColor(Color.black);
g.fillOval(130,220,20,20);
}
}
class Bubble extends Thread { //定义泡泡类.
Fish fish = null;
int x,y,ySpeed;
public void draw(Graphics g2) { //画泡泡.
g2.setColor(Color.grey);
g2.fillOval(x,y,20,20);
g2.setColor(Color.white);
g2.fillOval(x+2,y+2,16,16);
}
public Bubble (int _x,int _y,int _ySpeed) {
x = _x;
y = _y;
ySpeed = _ySpeed;
}
public void run() {
while(true) {
y += ySpeed;
try
{sleep(30);}
catch(InterruptedException ie)
{System.err.println("Thread interrupted");}
fish.repaint();
}
}
}
public class Fish_bubble {
public static void main(String args[])
{new Fish();}
}
追问:你好。能否改成点了"吐泡泡"之后,每隔1,2秒自动吐一个泡泡,而不是点一下,出一个泡泡。
答案:您好,您的程序总体是写的不错的,但很多地方仍有问题,根据您的要求我重新写了一个鱼吐泡泡的程序,在您原来的基础上有较大改动,必要的地方都做了注释,希望对您有所帮助,代码如下:
--程序截图--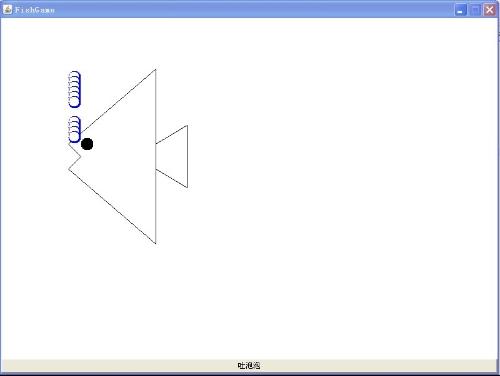
--java 代码--
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.awt.color.*;
import java.util.Vector;
import java.util.Iterator;
class Fish{//鱼类
public void draw(Graphics g) {//画鱼
Color c = g.getColor();
g.setColor(Color.black);
g.drawLine(110,230,250,110);
g.drawLine(110,270,250,390);
g.drawLine(110,230,130,250);
g.drawLine(110,270,130,250);
g.drawLine(250,230,300,200);
g.drawLine(250,270,300,300);
g.drawLine(300,200,300,300);
g.drawLine(250,110,250,390);
g.setColor(Color.black);
g.fillOval(130,220,20,20);
g.setColor(c);
}
}
class Bubble{//泡泡类
int x=110,y=233,x_speed,y_speed;//泡泡的位置以及x,y方向的移动速度
public void draw(Graphics g){//画泡泡
Color c= g.getColor();
g.setColor(Color.blue);
g.fillOval(x, y, 20, 20);
g.setColor(Color.white);
g.fillOval(x+1, y+1, 16, 16);
g.setColor(c);//把画笔默认颜色还原
y-=8;
}
}
public class FishGame extends Frame implements ActionListener{//窗体类
Vector<Bubble> bubb = new Vector<Bubble>();//每吐出的泡泡就添加进Vector集合
Image offScrrenImage = null;//重绘泡泡时消除上一次的轨迹,避免形成连续轨迹
Fish fish = new Fish();
public FishGame(){
this.setLayout(new BorderLayout());
this.setTitle("FishGame");
this.setBackground(Color.white);
this.setSize(800,600);
this.setResizable(false);
this.setVisible(true);
this.addWindowListener(new WindowAdapter(){
public void windowClosing(WindowEvent e){
System.exit(0);
}
});
Button b = new Button("吐泡泡");
b.addActionListener(this);
this.add(b,BorderLayout.SOUTH);
Thread t = new Thread(new BubbleThread());
t.start();
}
public void paint(Graphics g){
fish.draw(g);
Iterator it = bubb.iterator();
while(it.hasNext()){
((Bubble) it.next()).draw(g);
}
}
public void update(Graphics g){//绘制虚拟图片
if(offScrrenImage == null){
offScrrenImage = this.createImage(800,600);//设置虚拟图片和主窗体背景一样宽
}
Graphics goffScreen = offScrrenImage.getGraphics();//拿到虚拟图片的画笔
Color c = goffScreen.getColor();
goffScreen.setColor(Color.white);
goffScreen.fillRect(0, 0, 800, 600);
paint(goffScreen);
g.drawImage(offScrrenImage, 0, 0, null);
goffScreen.setColor(c);
}
public void actionPerformed(ActionEvent arg0) {
bubb.add(new Bubble());
}
private class BubbleThread implements Runnable{//控制泡泡移动的线程类
public void run() {
while(true) {
try
{
Thread.sleep(300);
}
catch(InterruptedException e){
e.printStackTrace();
}
repaint();
}
}
}
public static void main(String args[]){
new FishGame();
}
}
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.*;
class Fish extends Frame implements ActionListener {
Button start = new Button("吐泡");
// fish[] = new Fish[5];
Bubble []bubble=new Bubble[1];//在此设置吐泡的数量
public Fish() {
super("Fish");
add(start);
start.addActionListener(this);
setSize(500,500);
setVisible(true);
setLayout(new FlowLayout());
validate();
addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent we){
System.exit(0);
}
});
}//缺少一个"}"
public void actionPerformed(ActionEvent ae) {
//for(int i=0;i<fish.length;i++) {
//定义泡泡速度和坐标.
int ySpeed = 20;
int x = 100;
int y = 255;
//实例化线程.
// fish[i] = new Fish(x,y,ySpeed);
//fish[i].start();
// }
for(int i=0;i<bubble.length;i++){
bubble[i]=new Bubble(x,y,ySpeed,this);
bubble[i].start();
}
}
//public void paint(Graphics g2) {
//for(int i=0;i<fish.length;i++)
//if(fish[i] != null)
//fish[i].draw(g2);
//}
// }
//画鱼.
public void paint(Graphics g) {
g.drawLine(110,230,250,110);
g.drawLine(110,270,250,390);
g.drawLine(110,230,130,250);
g.drawLine(110,270,130,250);
g.drawLine(250,230,300,200);
g.drawLine(250,270,300,300);
g.drawLine(300,200,300,300);
g.drawLine(250,110,250,390);
g.setColor(Color.black);
g.fillOval(130,220,20,20);
}
}
class Bubble extends Thread { //定义泡泡类.
Fish fish = null;
public int x,y,ySpeed;
public int srcx,srcy;//当泡到达窗口顶部是,x,y置为此初始值
Graphics g=null;
public void draw(Graphics g2) { //画泡泡.
//定义两个随机变量,用于泡泡的水平位置
if(y<200){
double tempx1=Math.random()*(5);
double tempx2=Math.random()*(-5);
x+=(tempx1+tempx2);
System.out.println("tempx1:"+tempx1+",tempx2:"+tempx2);
}
g2.setColor(Color.gray);
g2.fillOval(x,y,20,20);
g2.setColor(Color.white);
g2.fillOval(x+2,y+2,16,16);
 
上一个:JAVA语言设计实验,帮忙解决追加100分
下一个:用Java写一个计算机的程序怎么写啊