C++中的优先级队列使用范例
[cpp]/*
This is a free Program, You can modify or redistribute it under the terms of GNU
*Description:优先级队列使用范例
*Language: C++
*Development Environment: VC6.0
*Author: Wangzhicheng
*E-mail: 2363702560@qq.com
*Date: 2012/10/18
*/
/*
priority_queue 优先级队列是一个拥有权值概念的单向队列queue,
在这个队列中,所有元素是按优先级排列的。
在STL的具体实现中,priority_queue也是以别的容器作为底部结构,再根据堆的处理规则来调整元素之间的位置
*/
#include <iostream>
#include <queue>
#include <string>
using namespace std;
const int max=1000;
class Person {
private:
int nice; //优先级
string name;
public:
Person(int nice=0,string name="") {
this->nice=nice;
this->name=name;
}
Person(const Person& p) {
nice=p.nice;
name=p.name;
}
Person& operator=(const Person& p) {
nice=p.nice;
name=p.name;
return *this;
}
friend bool operator<(const Person &p1,const Person &p2) {
if(p1.getNice()!=p2.getNice()) {
return p1.getNice() < p2.getNice();
}
return p1.getName() < p2.getName();
}
void setNice(int nice) {
this->nice=nice;
}
int getNice() const {
return nice;
}
void setName(string name) {
this->name=name;
}
string getName() const {
return name;
}
friend istream& operator >>(istream& is,Person &p) {
int nice;
string name;
cout<<"请输入人员的优先级:";
cin>>nice;
if(!cin.good()) {
cerr<<"输入错误!"<<endl;
is.clear();
return is;
}
if(nice<0 || nice>=max) {
cerr<<"输入范围错误!"<<endl;
is.clear();
return is;
}
cout<<"请输入人员的姓名:";
cin>>name;
p.setNice(nice);
p.setName(name);
return is;
}
friend ostream& operator <<(ostream &os,Person &p) {
os<<"姓名:"<<p.getName()<<endl;
os<<"优先级:"<<p.getNice()<<endl;
return os;
}
};
class LessThan {
public:
bool operator()(const Person &p1,const Person &p2) {
if(p1.getNice()!=p2.getNice()) {
return p1.getNice() < p2.getNice();
}
return p1.getName() < p2.getName();
}
};
const int N=4;
void main() {
int nice;
string name;
int i;
Person p;
priority_queue<Person,vector<Person> >q;
for(i=0;i<N;i++) {
cout<<"请输入第"<<i<<"个人员信息"<<endl;
cin>>p;
q.push(p);
}
while(q.empty()==false) {
cout<<q.top();
q.pop();
}
}
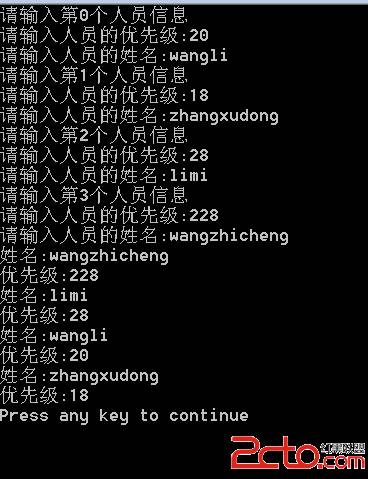
补充:软件开发 , C++ ,