Android 类似电影倒计时效果(两种随你选)
最近项目用到了这个功能,就实现了一下。有需要的朋友可以作为参考看一下,还是一个demo,如果添加到自己的项目中,最好改动美化一下。 先说一下第一种,也是个人倾向的一中,是用Activity加并设置style为Theme.Dialog。这样做是为了看起来悬浮在上面。效果如下: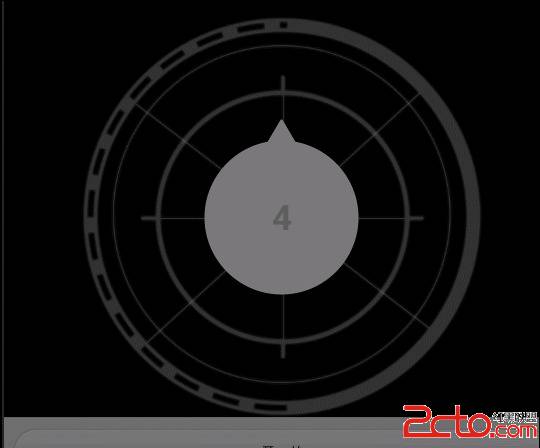
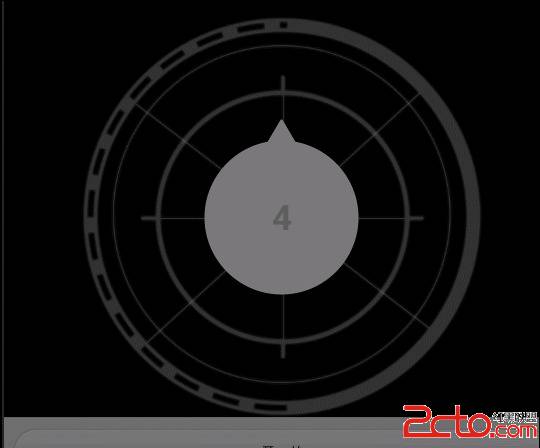
思路:主要就是利用动画来实现的,让指针图片不停的旋转,形成像电影倒计时效果。
代码:
1、Activity
import java.util.Timer; import java.util.TimerTask; import android.annotation.SuppressLint; import android.app.Activity; import android.os.Bundle; import android.os.Handler; import android.os.Message; import android.view.View; import android.view.View.OnClickListener; import android.view.animation.Animation; import android.view.animation.RotateAnimation; import android.widget.Button; import android.widget.ImageView; import android.widget.TextView; public class Test extends Activity { private long mlCount = 50; private long mCount = 0; TextView tvTime; private Button startbuttondaoji; private Timer timer = null; private TimerTask task = null; private Handler handler = null; private Message msg = null; private ImageView min_progress, min_progress_hand; Animation rotateAnimation; float predegree = 0; boolean okclear = false; @SuppressLint("HandlerLeak") @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); init(); } private void init() { setContentView(R.layout.test); tvTime = (TextView) findViewById(R.id.duocitvTime); startbuttondaoji = (Button) findViewById(R.id.startbuttonduoci); min_progress = (ImageView) this.findViewById(R.id.duocimin_progress); min_progress_hand = (ImageView) this .findViewById(R.id.duocimin_progress_hand); tvTime.setText("4"); SaveRun.setisjishi(false); handler = new Handler() { @Override public void handleMessage(Message msg) { switch (msg.what) { case 1: if(mlCount>1){ mlCount--; mCount++; }else{ break; } int totalSec = 0; totalSec = (int) (mlCount / 10); int sec = (totalSec % 60); try { rotateAnimation = new RotateAnimation(predegree, (float) (36 * mCount), Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f); rotateAnimation.setDuration(100); rotateAnimation.setFillAfter(false); min_progress_hand.startAnimation(rotateAnimation); min_progress.startAnimation(rotateAnimation); tvTime.setText(String.format("%1$2d", sec)); predegree = (float) (36 * mCount); } catch (Exception e) { tvTime.setText( sec +""); e.printStackTrace(); } break; default: break; } super.handleMessage(msg); } }; } @Override protected void onStart() { star(); super.onStart(); } private void star() { startbuttondaoji.setOnClickListener(new OnClickListener() { @Override public void onClick(View arg0) { startbuttondaoji.setVisibility(View.GONE); if (null == timer) { if (null == task) { SaveRun.setisjishi(true); okclear = false; min_progress.setVisibility(View.VISIBLE); task = new TimerTask() { @Override public void run() { if (null == msg) { msg = new Message(); } else { msg = Message.obtain(); } msg.what = 1; handler.sendMessage(msg); } }; } timer = new Timer(true); timer.schedule(task, 100, 100); } } }); } View.OnClickListener startPauseListener = new View.OnClickListener() { @Override public void onClick(View v) { if (null == timer) { if (null == task) { SaveRun.setisjishi(true); okclear = false; min_progress.setVisibility(View.VISIBLE); task = new TimerTask() { @Override public void run() { if (null == msg) { msg = new Message(); } else { msg = Message.obtain(); } msg.what = 1; handler.sendMessage(msg); } }; } timer = new Timer(true); timer.schedule(task, 100, 100); } else { try { SaveRun.setisjishi(false); okclear = true; task.cancel(); task = null; timer.cancel(); timer.purge(); timer = null; handler.removeMessages(msg.what); } catch (Exception e) { e.printStackTrace(); } } } };
}
2、布局文件
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/background_dark" >
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_above="@+id/buttonlinear" >
<ImageView
android:id="@+id/duocimin_dial"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:src="@drawable/iv_bg" />
<ImageView
android:id="@+id/duocimin_progress"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_cen补充:移动开发 , Android ,